package com.view.web.webview; import android.app.Activity; import android.content.Context; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; public class MainActivity extends Activity { private Button button; public void onCreate(Bundle savedInstanceState) { final Context context = this; super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); button = (Button) findViewById(R.id.buttonUrl); button.setOnClickListener(new OnClickListener() { @Override public void onClick(View arg0) { Intent intent = new Intent(context, WebViewActivity.class); startActivity(intent); } }); } }
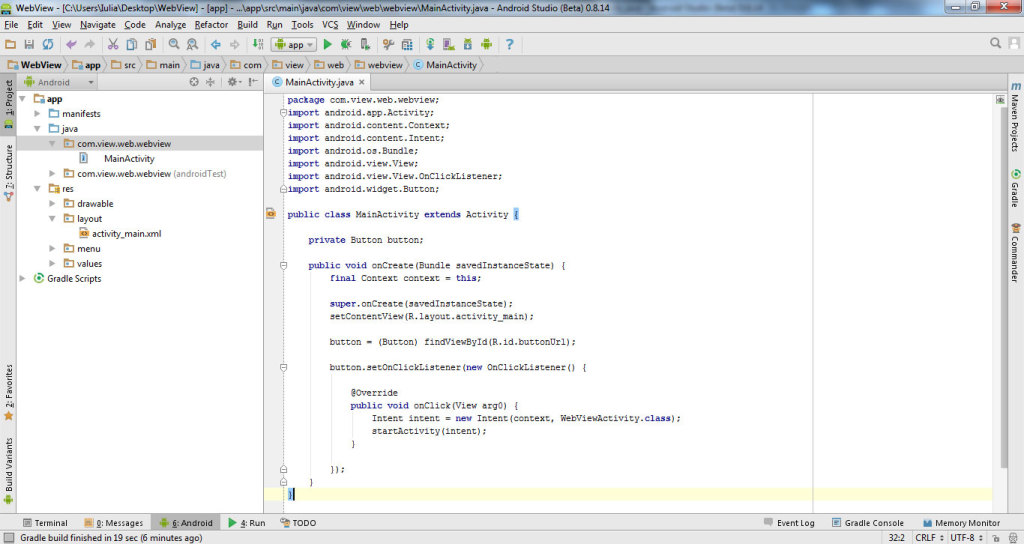
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <Button android:id="@+id/buttonUrl" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/red_button" android:textColor="#ffffff" android:textSize="20dp" android:text="GO TO android-coffee.com" /> </RelativeLayout>
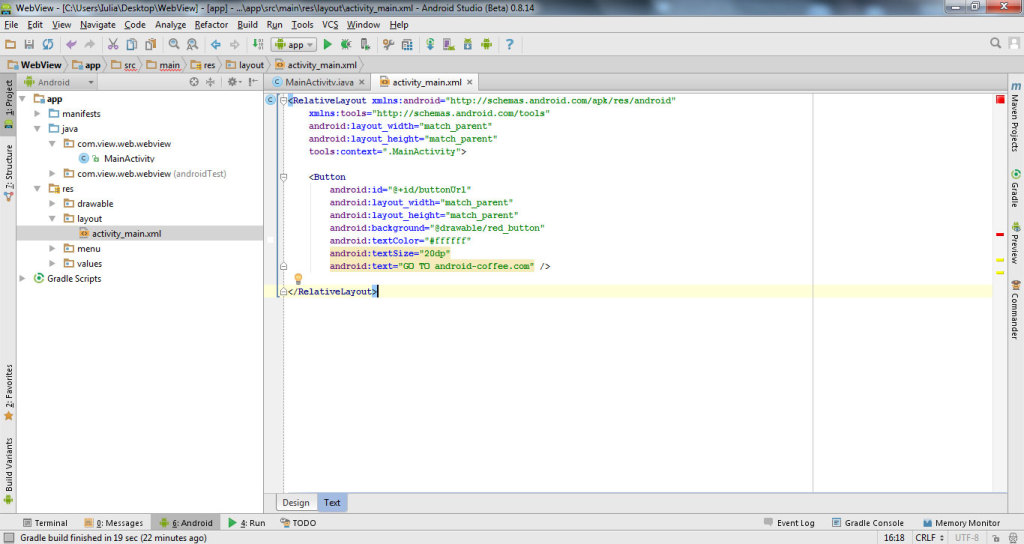
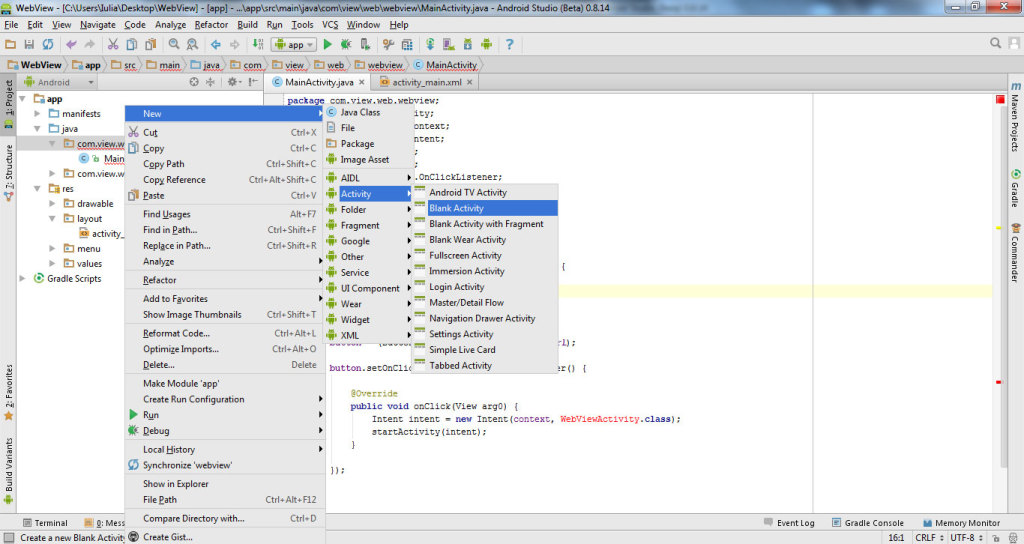
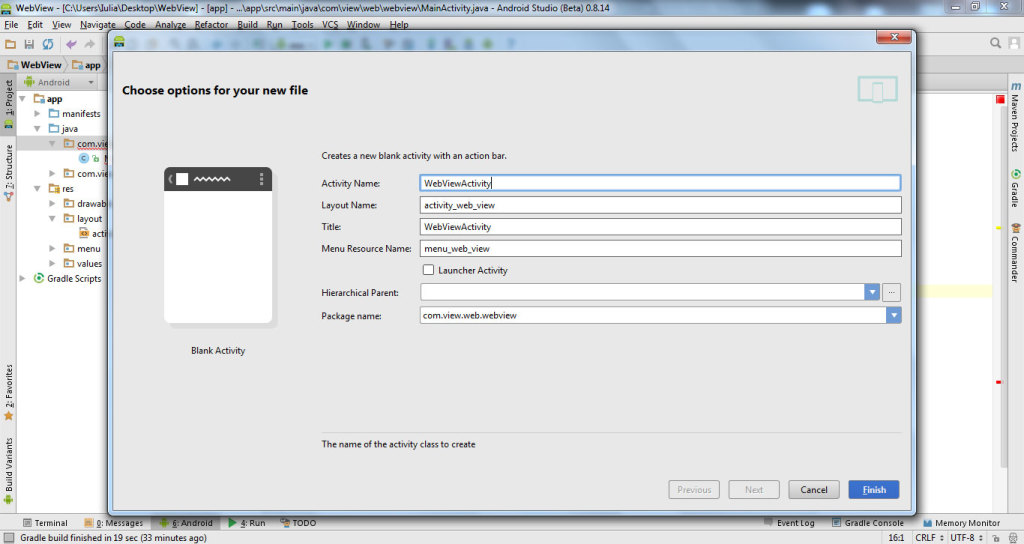
package com.view.web.webview; import android.app.Activity; import android.os.Bundle; import android.webkit.WebView; public class WebViewActivity extends Activity { private WebView webView; public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_web_view); webView = (WebView) findViewById(R.id.webView1); webView.getSettings().setJavaScriptEnabled(true); webView.loadUrl("https://android-coffee.com"); } }
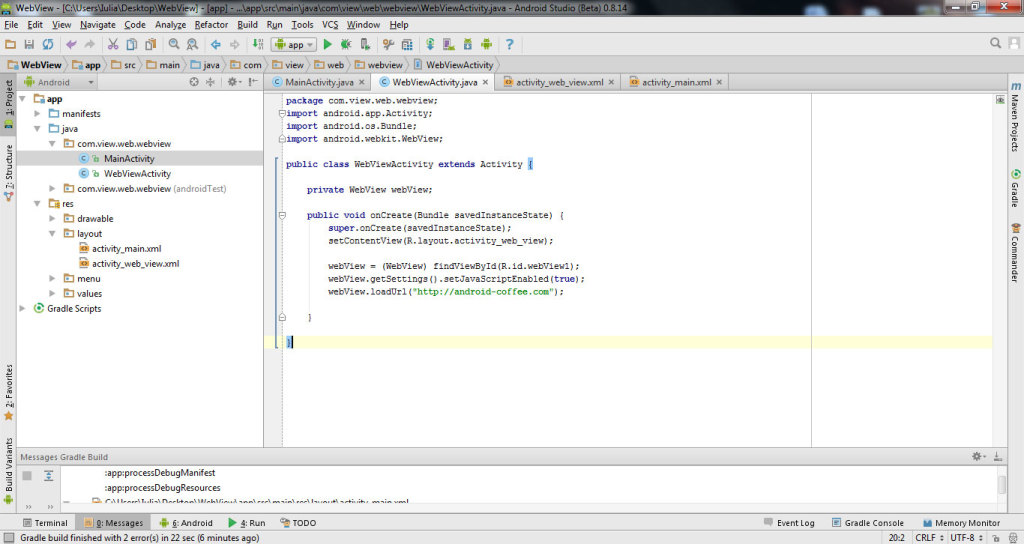
<?xml version="1.0" encoding="utf-8"?> <WebView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/webView1" android:layout_width="fill_parent" android:layout_height="fill_parent" />
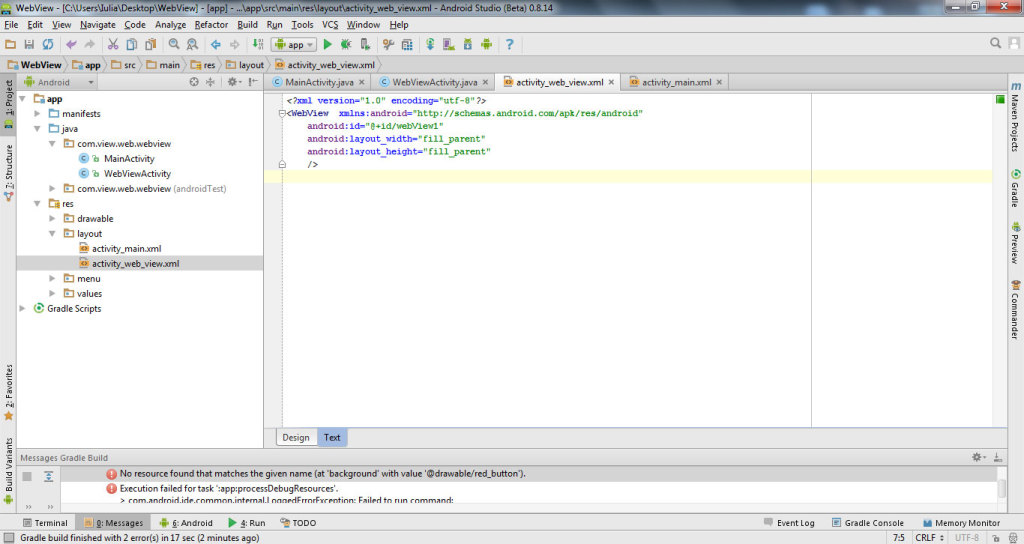
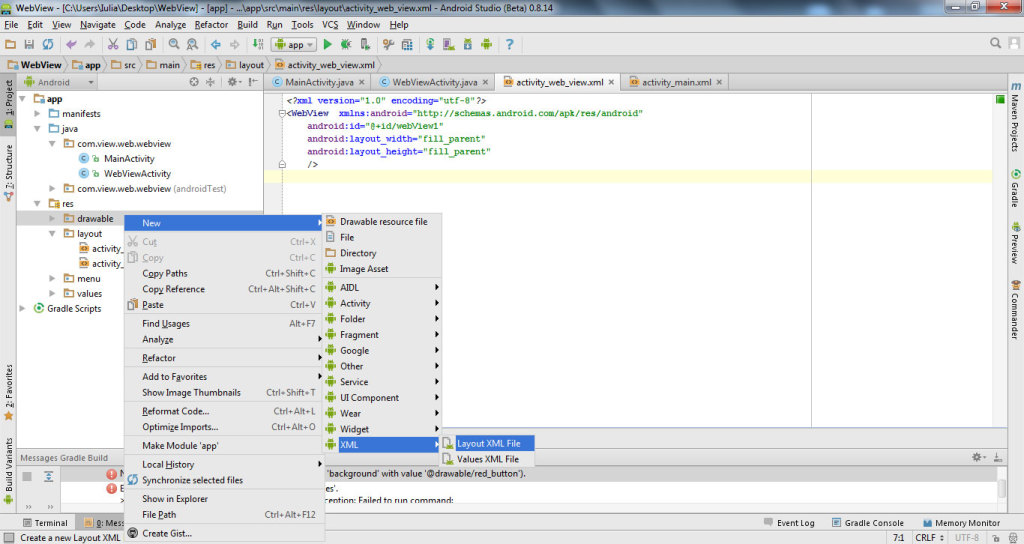
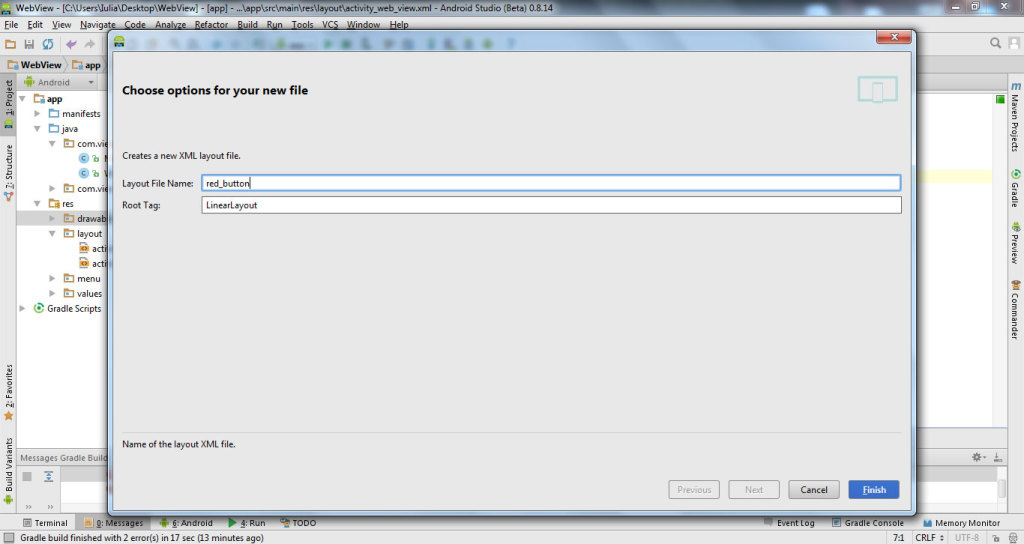
<?xml version="1.0" encoding="utf-8" ?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:state_pressed="true" > <shape> <solid android:color="#ef4444" /> <stroke android:width="1dp" android:color="#000000" /> <corners android:radius="6dp" /> <padding android:left="10dp" android:top="10dp" android:right="10dp" android:bottom="10dp" /> </shape> </item> <item> <shape> <gradient android:startColor="#ef4444" android:endColor="#000000" android:angle="270" /> <stroke android:width="1dp" android:color="#992f2f" /> <corners android:radius="6dp" /> <padding android:left="10dp" android:top="10dp" android:right="10dp" android:bottom="10dp" /> </shape> </item> </selector>
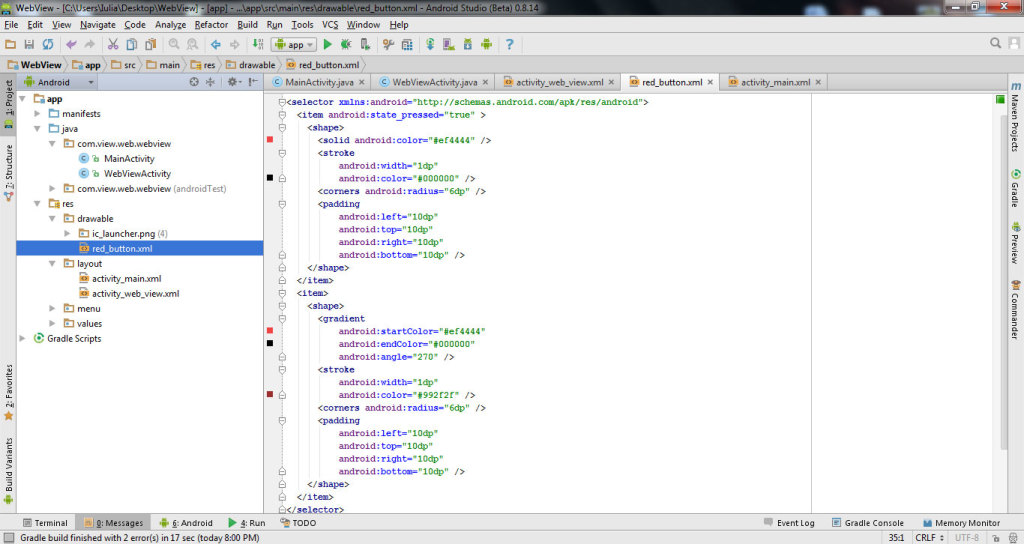
<uses-permission android:name="android.permission.INTERNET" />
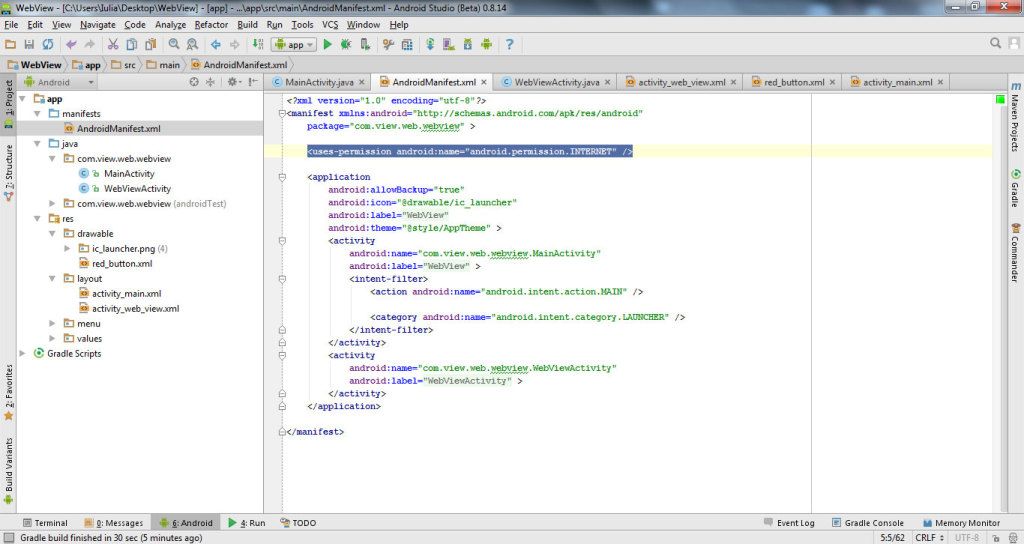
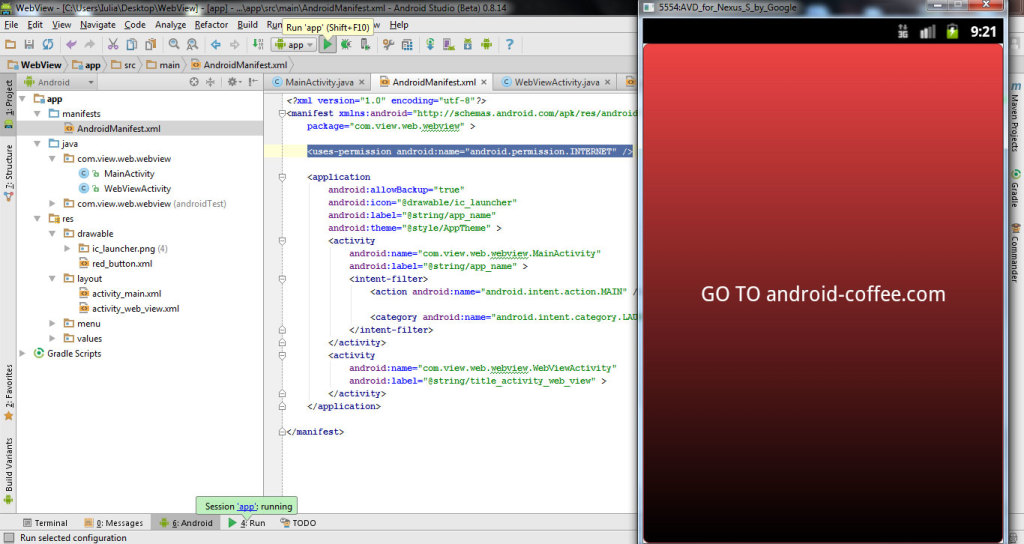
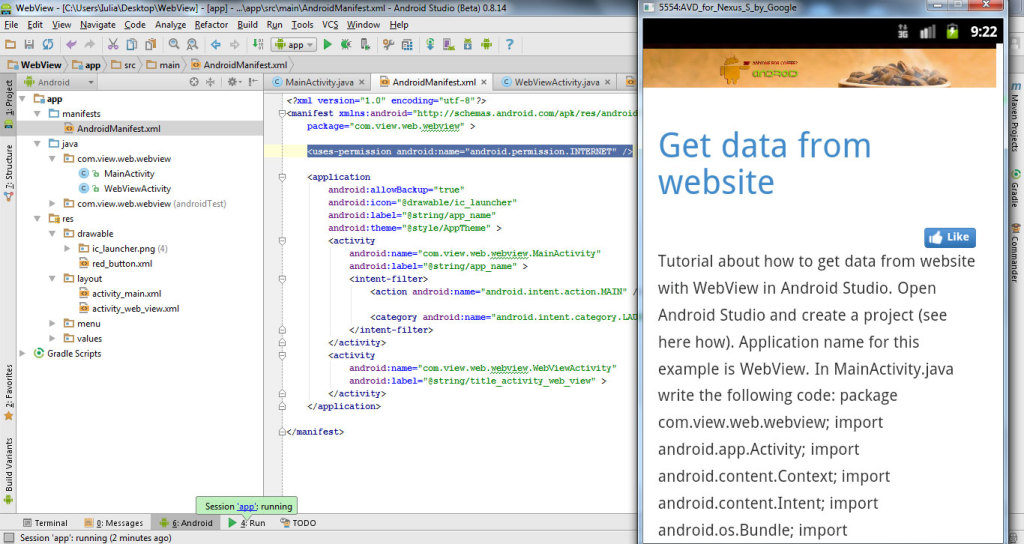
For further questions leave a message.
Why all people doing video-tutorials online aren’t doing it in the way you are? In a few minutes, I learned and was able to test what you are teaching. If you had spoken in the video, I think it would have lasted about 15 or 20 ‘boring’ minutes, besides you shared the code in your site. I’ve been watching tutorials on youtube for about 4 hours , and nobody else is doing it. It is really appreciated.
With the only thing I had issues to debug the project, was with this sentence in the activity_main.xml file:
minutes.android:background="@drawable/red_button"
Android Studio 1.0.1 says: “Rendering problems. Couldn’t resolve resource @drawable/red_button (2 similar errors not shown). Failed to convert @drawable/red_button into a drawable”.
So I changed it for a red color: #F00, and it worked.
Thanks again for this practical and fun tutorial. I hope you keep doing more.
Thanks for your feedback!
What if we wanted to use the data from website as a string?
Webview is used to just show the data (As in a browser) but I need to use the data as a string in my app. How can I do that?
Good work. My question is how to create menu buttons in webview app ? for example I want my web to open in webview and display particular menu buttons . User click on his choice of buttons ? one more thing I am very new to all this language game.
Thanks dear for such a great share of knowledge.
your way of help to learner as snap shoot and code provided is vary interested.
Thank you 🙂
I need to access one particular field (example petrol price from a website) to my app…
plzz help me
is there a way to pull a specific data from the website, like content of a div?
You’ll probably be able to do it using the DOM via a class or id for the div itself. Here’s the w3schools page in case that helps:
http://www.w3schools.com/jsref/dom_obj_div.asp
God Bless You
Nice one…
Thanks, that is extremely helpful. I have been trying to work this out for a new app I am creating for my website. Extremely well laid out tutorial.
Thanks for sharing.
Hi – this is well laid out, and very helpful.
Thank you.
Gary
Nice Work!
I am thinking of learning how to retrieve data from website and use it on the android app.this was helpful for me in taking the first step in the process.
Can you suggest me how to get more info about retrieving data from websites?
of all i admire you and the one other on php pot you have given most precise and dynamic way of teaching.thanks god for giving you 2 humans.
Here i got a confusion in url how can I add search bar to search a specific text which I want to get from a website know more my website.